Displaying a featured image when hovering over a post link is a great way to enhance user experience and provide a visual preview of your content. In this tutorial, we’ll go step by step to achieve this effect using WordPress functions, HTML, CSS, and finally, we’ll show how to implement it as a shortcode.
Experience lightning-fast speed, rock-solid security, and world-class support tailored for WordPress. Simply better hosting.
How to display feautured image of a post when hovering on the posts link
Step 1: Fetching the Featured Image
Each WordPress post has a featured image, also known as a post thumbnail. To retrieve it, we use the get_the_post_thumbnail()
function.
Basic Code to Get Featured Image
2 3 4 |
<?php echo get_the_post_thumbnail(get_the_ID(), 'medium'); ?> |
This function fetches the featured image of a post in the ‘medium’ size.
Suggested Read: Track and Display ‘Add to Cart’ Button Click Counts in WooCommerce
Step 2: Displaying Post Titles with Featured Images
Now, let’s create a simple HTML structure where post titles are displayed as links, and the featured image appears on hover.
Add This Code to Your Theme File
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<ul class="featured-posts-list"> <?php $args = array( 'post_type' => 'post', 'posts_per_page' => 10, ); $query = new WP_Query($args); if ($query->have_posts()) : while ($query->have_posts()) : $query->the_post(); ?> <li class="featured-post-item"> <a href="<?php the_permalink(); ?>" class="post-link"><?php the_title(); ?></a> <div class="featured-image-preview"> <?php echo get_the_post_thumbnail(get_the_ID(), 'medium'); ?> </div> </li> <?php endwhile; wp_reset_postdata(); endif; ?> </ul> |
In the above code:
- Fetches the latest 10 posts.
- Displays each post title as a link.
- Includes a hidden
div
that contains the featured image
Step 3: Adding CSS for the Hover Effect
To make the featured image appear only when hovering over the title, add this CSS to your theme’s style.css
file:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
.featured-posts-list { list-style: none; padding: 0; } .featured-post-item { position: relative; margin-bottom: 10px; } .post-link { text-decoration: none; color: #0073aa; font-weight: bold; } .featured-image-preview { display: none; position: absolute; top: 100%; left: 0; width: 200px; background: #fff; border: 1px solid #ddd; padding: 5px; box-shadow: 2px 2px 10px rgba(0, 0, 0, 0.2); z-index: 9999; } .featured-post-item:hover .featured-image-preview { display: block; } |
This CSS ensures that the featured image appears when hovering over the post title.
Complete Code: Implementing the Code as a Shortcode
For easier use, we can convert this into a shortcode so it can be placed anywhere on the site.
Add This Code to Your functions.php
File
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 |
function featured_posts_hover_shortcode($atts) { ob_start(); // Start output buffering // Parse shortcode attributes (default: show 10 posts) $atts = shortcode_atts( array( 'posts_per_page' => 10, ), $atts, 'featured_posts_hover' ); // Fetch latest posts $args = array( 'post_type' => 'post', 'posts_per_page' => intval($atts['posts_per_page']), ); $query = new WP_Query($args); // Add inline CSS (loads only when shortcode is used) ?> <style> .featured-posts-list { list-style: none; padding: 0; } .featured-post-item { position: relative; margin-bottom: 10px; } .featured-post-item a { text-decoration: none; color: #0073aa; font-weight: bold; } .featured-post-item:hover a { color: #005177; } .featured-image-preview { display: none; position: absolute; top: 100%; left: 0; width: 200px; background: #fff; border: 1px solid #ddd; padding: 5px; box-shadow: 2px 2px 10px rgba(0, 0, 0, 0.2); z-index: 9999; } .featured-post-item:hover .featured-image-preview { display: block; } </style> <?php if ($query->have_posts()) : echo '<ul class="featured-posts-list">'; while ($query->have_posts()) : $query->the_post(); $thumbnail = get_the_post_thumbnail(get_the_ID(), 'medium'); // Get featured image HTML ?> <li class="featured-post-item"> <a href="<?php the_permalink(); ?>"><?php the_title(); ?></a> <div class="featured-image-preview"> <?php echo $thumbnail; ?> </div> </li> <?php endwhile; echo '</ul>'; wp_reset_postdata(); else : echo '<p>No posts found.</p>'; endif; return ob_get_clean(); // Return buffered content } add_shortcode('featured_posts_hover', 'featured_posts_hover_shortcode'); |
Now, you can use this shortcode on any post or page:
2 3 4 |
[featured_posts_hover] |
To customize the number of posts:
2 3 4 |
[featured_posts_hover posts_per_page="5"] |
Output: View Demo
-
PhonePe Standard Checkout – Authorization Token Generation in PHP
-
Vibe Coding in 2025: Will Developers Still Need to Code?
-
WordPress parent company Automattic layoffs 16% of its workforce
-
Top AI Tools to Automate Business Operations
-
The Future of Money: A Beginner’s Guide to Bitcoin and Cryptocurrency
Are you in need of a skilled WordPress developer to bring your website vision to life?
Look no further! Whether you need custom themes, plugin development, site optimization, or ongoing support, I offer expert WordPress development services to suit your needs.
Conclusion
Adding a hover effect to display featured images in WordPress is a simple yet effective way to enhance user experience. We first created a step-by-step implementation using PHP and CSS. Then, we converted the code into a reusable shortcode. Now, you can easily add this feature anywhere on your WordPress site to enhance user engagement.
This approach is lightweight, does not rely on additional plugins, and keeps your website optimized. Try implementing it on your site and make your post links more engaging!
Thanks for reading 🙏, I hope you found this tutorial helpful for your project. Keep learning! If you face any problems – I am here to solve your problems.
FAQs
Yes, you can modify the image size by changing 'medium'
to 'thumbnail'
, 'large'
, or a custom size in get_the_post_thumbnail()
.
Yes, you can replace 'post'
in the $args
array with your custom post type name.
Use the provided shortcode by adding it to a page, post, or widget.
No, this is a lightweight solution. However, if you display too many posts, it could slightly impact page load time. Optimize images for best performance.
Yes! Modify the CSS styles to change the position, animation, or transition effects for a more dynamic experience.
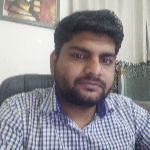
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co